Quit using your mouse - use IntelliJ shortcuts!
Thierry Gerritse - Aug 19, 2022
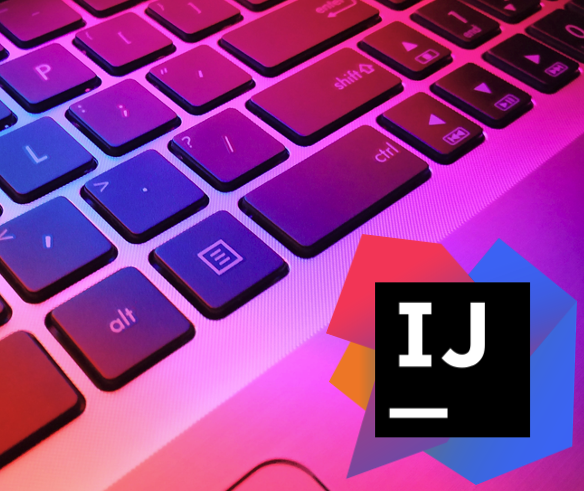
We all have our own preferred way of working, some of us use a lot of shortcuts, some use the mouse for everything. But simply stated, shortcuts are much faster. If you are looking to boost your productivity when coding, learning to use IntelliJ’s shortcuts might be a good start. In this article we will take a look at how you can use IntelliJ’s shortcuts to speed up your coding process. We’ll look into “Boilerplate” code generation, defining and easily working with variables, generating methods, quickly completing statements and more.
This article will focus on Java code, but many of the examples will also apply to other languages.
IntelliJ offers many shortcuts to help you, you can find them well documented here: IntelliJ IDEA Reference Card. There are however so many that it might be hard to pick which to memorize, therefor we will focus on a smaller selection. A lot of shortcuts exist to help you quickly search and navigate through your project. While those are certainly useful to learn about, we will instead be focussing on features that help you write and enhance your code. The shortcuts in this article are specified as the default macOS keymap but can be easily translated to Windows or Linus keymaps using the reference card.
“Boilerplate” code generation:
Let’s start with some basics to help write new code faster.
If it comes to writing new classes there are many ways to make your life easier. There are plugins to help you do this like Lombok and in some cases record classes will help. However, not everyone has the privilege to use Java 17 or those plugins. Fortunately, IntelliJ can also produce most of the “boilerplate code” for you.
We’ll take a new class as an example, class: Dog
in this class we define two fields: name
and age
. At this point IntelliJ can already generate a constructor, getters, setters, equals and hashcode methods and a toString method. By pressing CMD + N IntelliJ will show you a dialog (that you can easily complete without using your mouse) providing all options.
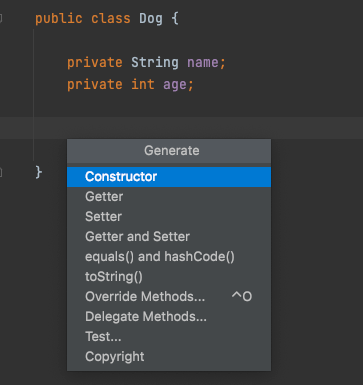
To make things even simpler, when you want to generate any of these but the constructor, you can just start typing, getN
or equ
and IntelliJ will suggest to generate the method for you.
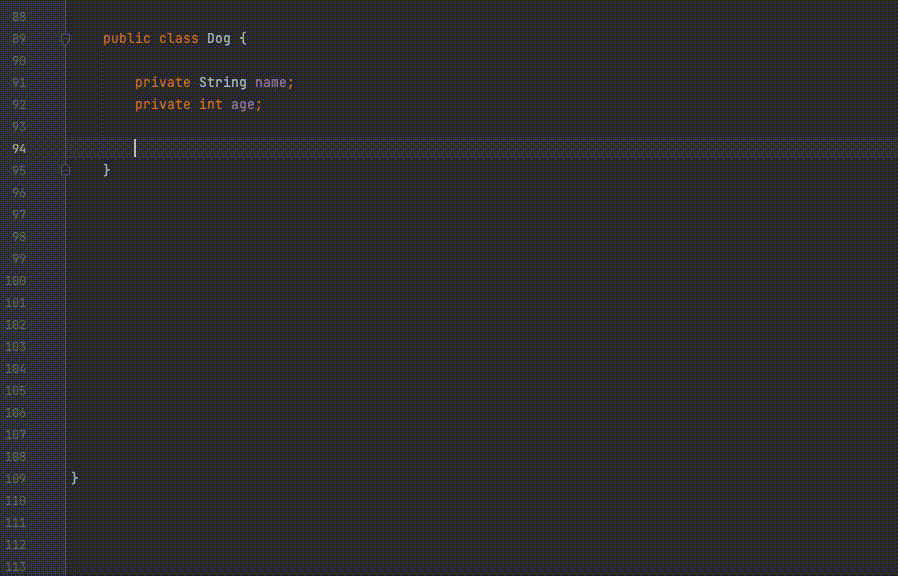
Easily defining variables:
Since most time is usually not spend writing classes, but actually using them we will now have a look at how IntelliJ can help you here.
IntelliJ will help you by saving time typing, for example if you have a long class name to spell out, you can do so by just using the upper-case letters, e.g. if you have a class named: ExampleClassWithExceptionallyLongName
, when you start typing: new ECWEL
IntelliJ will start showing suggestions and chances are the correct class will be right on top.
At that point it is just a matter of pressing enter once and the auto complete will do the rest.
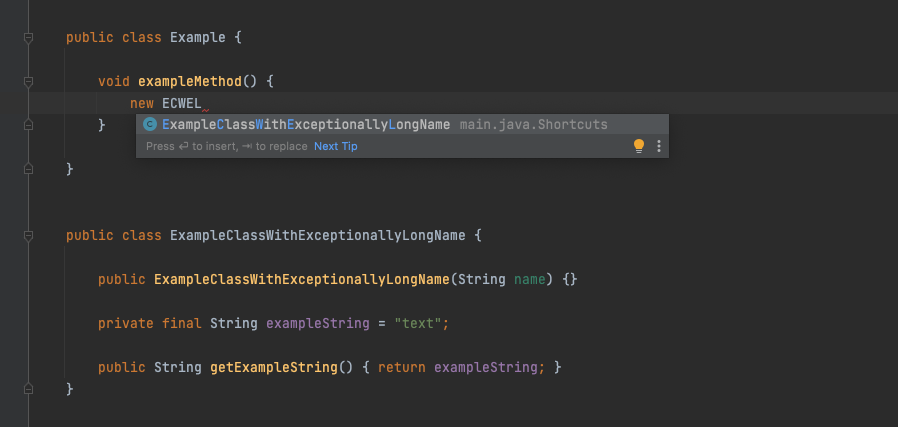
We can take it one step further: you can have IntelliJ help you define a variables. Following up on the scenario from before if we want to define a variable of the new class instance, just press Alt + Enter, Enter, and IntelliJ will create the variable for you. The variable is automatically named, so (most of the time) it saves you time of having to think of a name. The same works for calling methods on a class and defining variables out of the result.
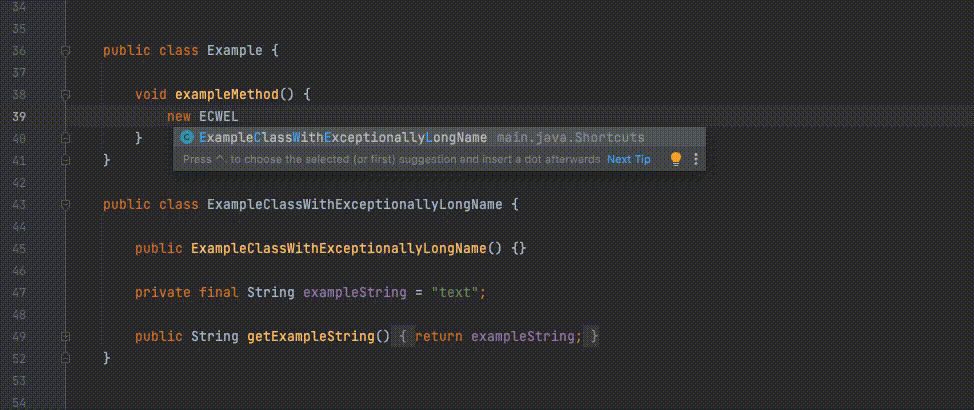
If you have a preference for old fashioned typed variables, you can configure IntelliJ to generate those instead of inferred vars.
As an alternative to using Alt + Enter to define a variable, you can follow up with CTRL + . and IntelliJ will finish your statement adding a dot at the end, allowing you to quickly select “var” from the auto suggestions to automatically define a variable for you.
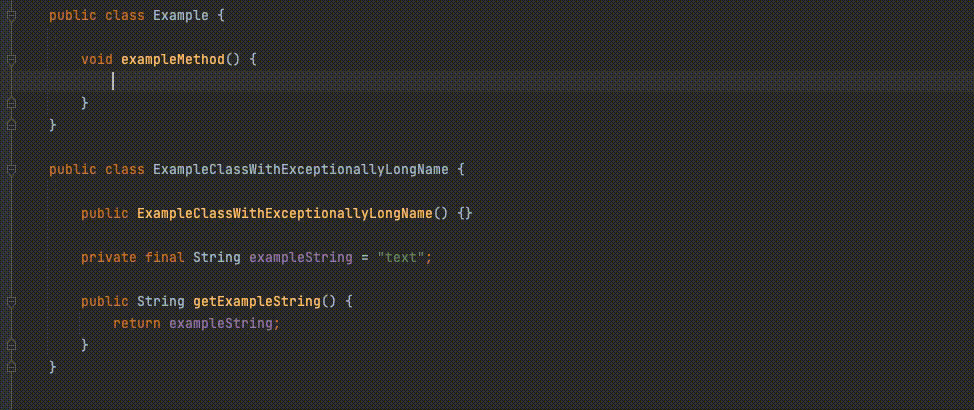
The power of the dot.
This follow up with a dot function as we have seen in the last paragraph is useful in many more situations. It can of course be used to display the exposed methods and attributes of a class, but it can do much more. As we mentioned earlier in the article, IntelliJ can generate a lot of things for you using insert. But by appending a dot behind a variable for example: .for + Enter behind a variable of an iterable type, will automatically wrap a generate a for loop for you.
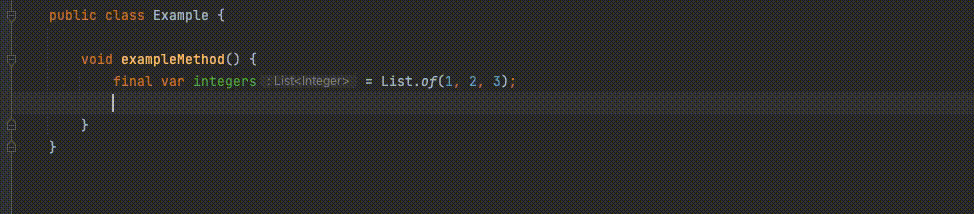
The same method works for a Boolean variables or expressions. By typing: .if + Enter, IntelliJ will automatically wrap it in an if-statement.
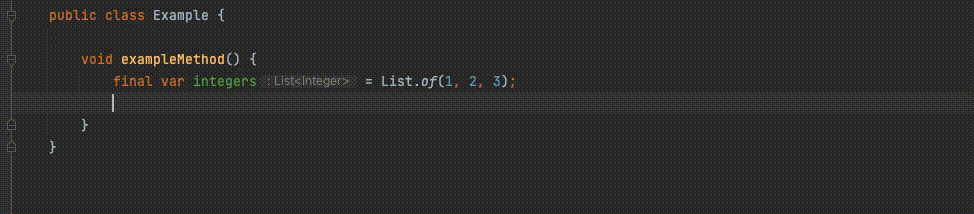
The dot will help you with a lot of other things such as returning the variable from a function, or defining a field, so be sure to try it and see all the options available to you!
Method generation and method calls:
Next up: calling methods. Writing out method calls can sometimes take a lot of time. IntelliJ can help you with this as well. You can use auto complete, but even simpler (similar to instantiating a class): you can simply start typing the first and capital letters of a method.
As an example, your class has a method: createSumOfTwoValues
, calling this method you can just type cs
and IntelliJ will already let you insert the options.
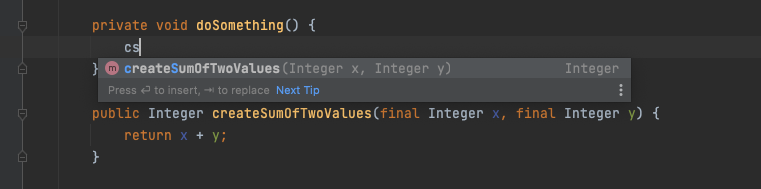
That may sound a bit simple, but it also works in depth: from within class Example
, you can call methods on class OtherExample
, using just some of the letters in the name. Like: oe.cs
.
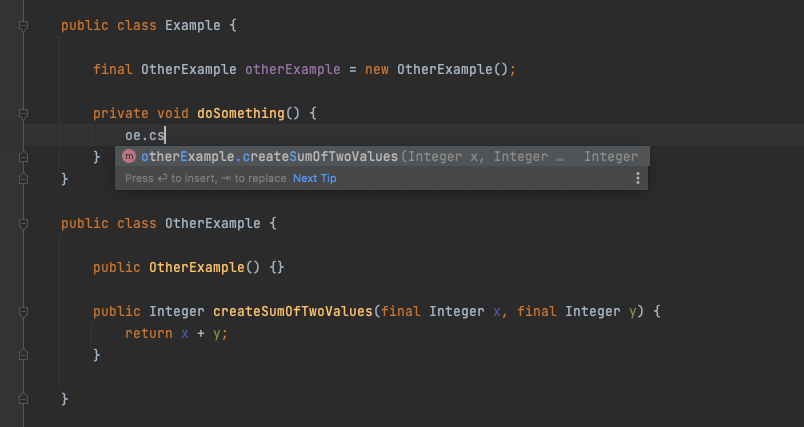
Another time consuming task related to method calls is having to refactor them. Looking at the previous example if I would want to change the method call from createSumOfTwoValues
to createModulusOfTwoValues
I have to delete the whole name, and enter it again. When using the standard auto complete it you will still have to delete the old method name.
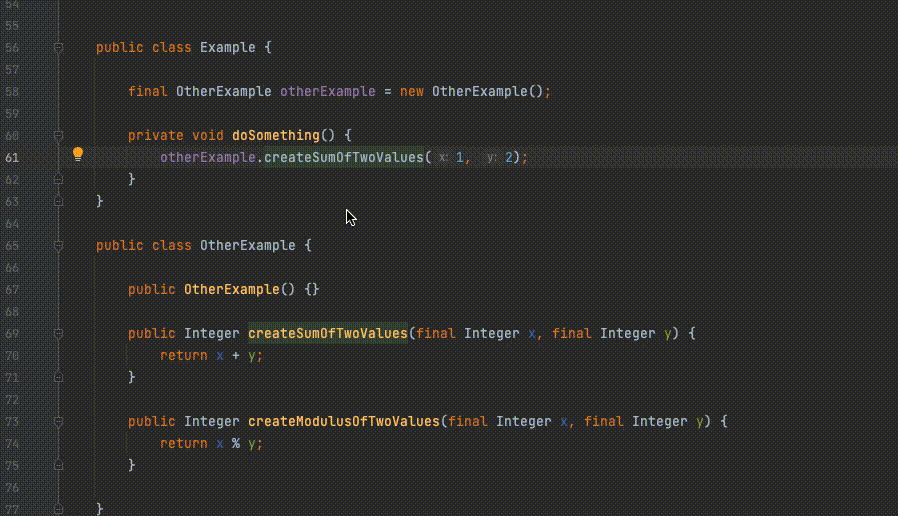
If you find yourself in this situation, try using tab instead of enter, and IntelliJ will replace the method call instead.
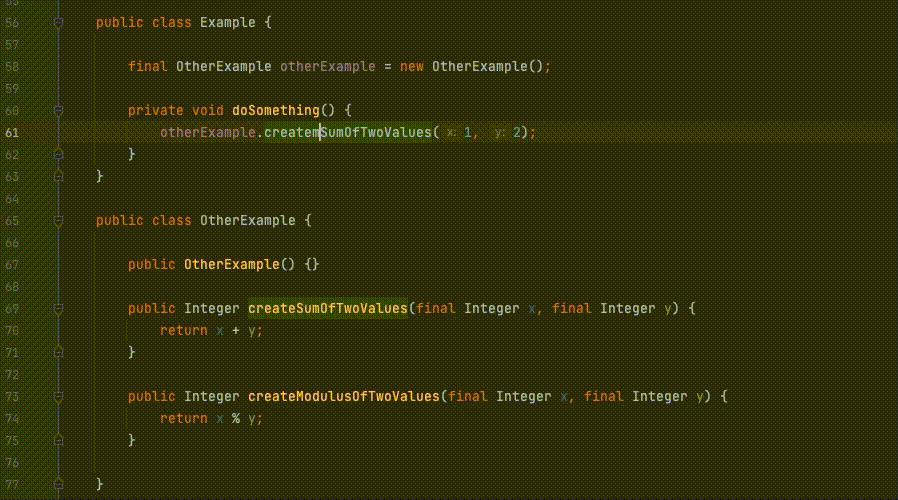
Resolving errors and warnings:
As you may have noticed IntelliJ will warn you of many code smells and errors when editing. Usually, you see a Red a Yellow or a Grey line under the affected line of code.
Most of the time IntelliJ has a suggestion to fix the issue as well. Let’s look at another example of how IntelliJ shortcuts can help your productivity.
An common situation where IntelliJ shows a red line, is while adding new methods to a class or new parameters to methods. It is possible to add or change the methods first and then start using them or update their usage. However, I find it easier and faster to start with the usage first. And that is something IntelliJ will make very easy for you.
If we look again at our example class, and we want to add a method, multiplyValues
to the OtherExample
class. It is possible to simply write out the name of the new method you wish to call, press Alt + Enter and let IntelliJ do the rest.
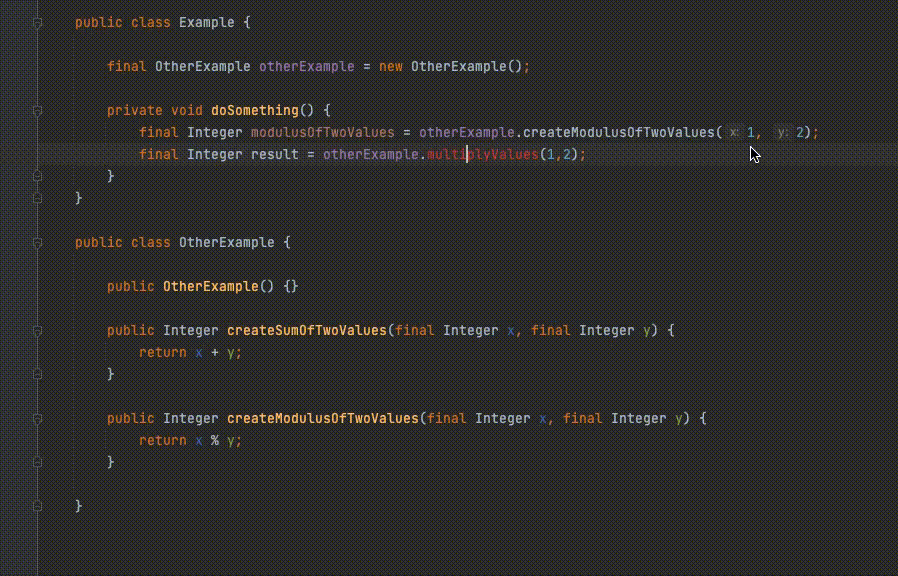
This also works when you want to add a new parameter to a method, e.g., let’s add a third parameter to the method we just created. Just by changing the usage with a third parameter pressing Alt + Enter and letting IntelliJ do the rest.
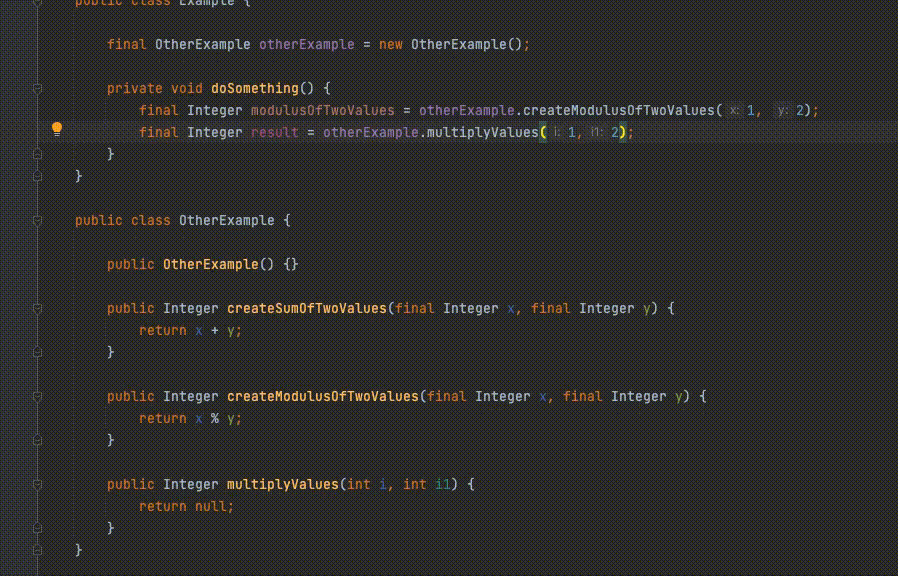
Usually when you see a red or yellow in IntelliJ, Alt + Enter will have a suggestion, and often those save you a lot of time. Make sure to try it as often as seems helpful!
Calling static methods:
IntelliJ can also help you use static methods.
As you have seen in the article so far, Alt + Enter is a go to shortcut, however, when working with static methods it is a bit harder. For example, if you want to use Pattern.matches()
somewhere in your project to evaluate a regular expression. You might try to type matches
and follow with Alt + Enter, IntelliJ will suggest several things such as generating a variable. But it will not find the method you’re looking for. Instead, when working with static methods, you can use CTRL + Space x 2 to find you a matching static method. Once more, simply press enter next and IntelliJ will do the work for you.
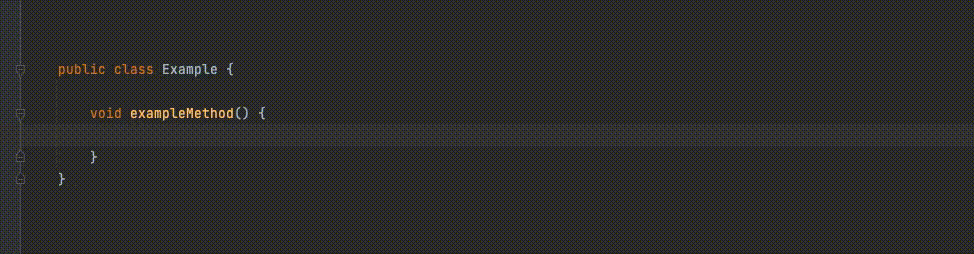
As an added bonus, when pressing Alt + Enter instead of Enter after selection the wanted option, IntelliJ will even suggest an automatic static import for you.
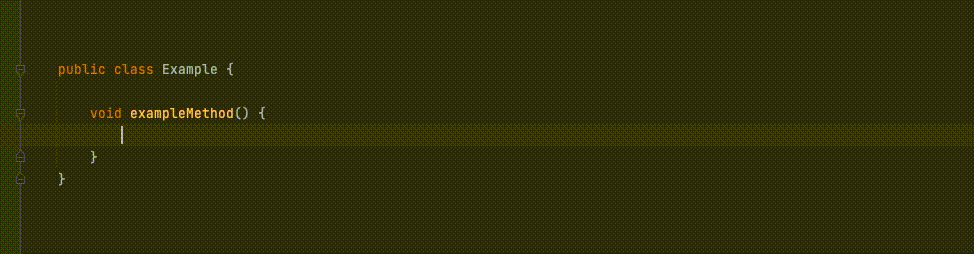
Completing statements:
As a Java developer you need to add a semicolon at the end of a statement to close it. However, your usual typing flow for a line of code does not go from left to right in one go, you have to navigate your cursor to the end at some point to close the statement. This is another example of a time-consuming practice that IntelliJ can help you with. When finished writing your statement, just press CMD + Shift + Enter, and IntelliJ will finish the statement for you, closing the line with a semicolon and even formatting it.
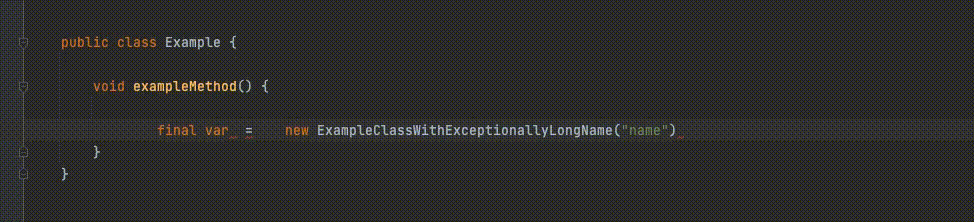
This great functionality works for finishing more than just a method call, for example it will also finish your if statement, or even the definition of a new method or class.
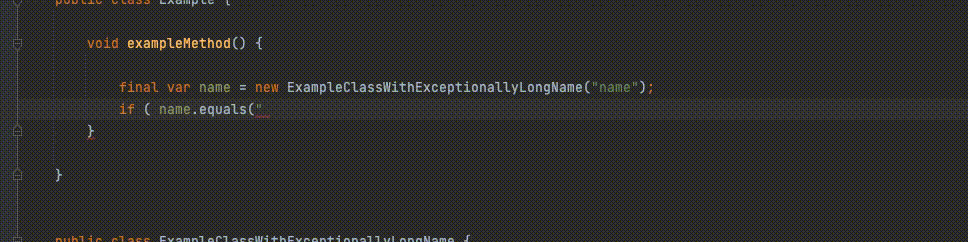
Smart code selection:
The last feature we will look into in this article is maybe one of the simplest and it will help you select code without using you mouse. Of course, it is possible to do this using Shift and CMD, Alt and the arrow keys, but IntelliJ has an even nicer option. With the cursor at any position by using Alt + Arrow key up, IntelliJ will expand the selection to the next syntax block. For example, when you are coding in a big method, and you are looking to make it smaller by splitting it up, IntelliJ can help you make a logical selection of a part of the method, just by making the selection bigger. It also makes the selection smaller when pressing Alt + Arrow key down.
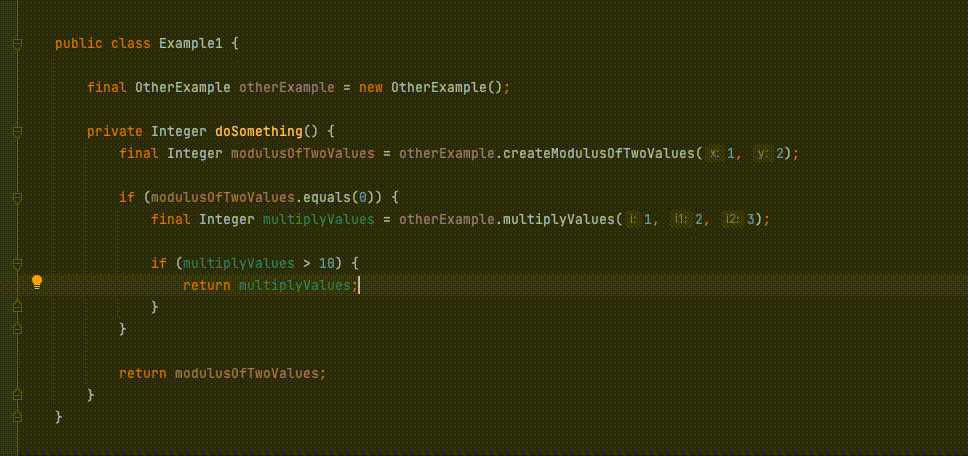
Closing remarks:
In the beginning the shortcuts might seem a bit overwhelming or confusing, but soon enough if will be almost automatic. To help you start an IntelliJ plugin called Key Promoter X exists to help you train with, and remember the shortcuts.
Whether you are just starting as a developer or trying to improve yourself hopefully this article has taught you something you did not yet know and can use to work more efficiently.